For AssetBundles to be available in BOLT you first have to add the proper option to the “Assembly Options” in the BOLT setup area.
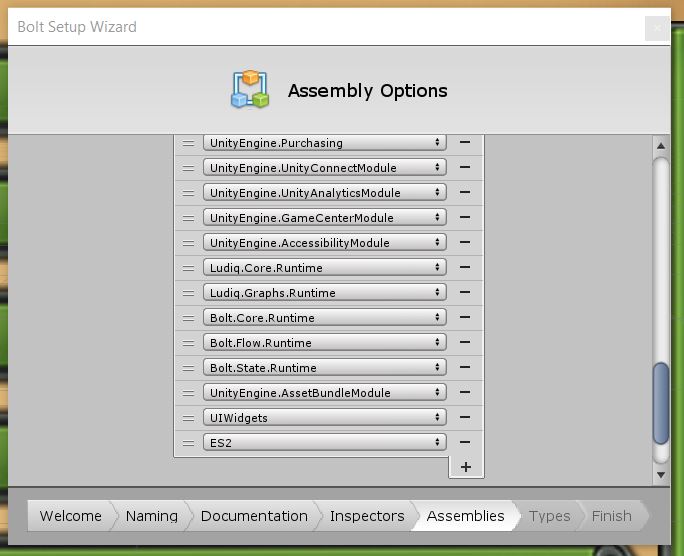
Loading AssetBundles
Loading Assets from a bundle in Unity to prevent the 5GB limit that the “Resource” folder puts on all project assets loaded during runtime.
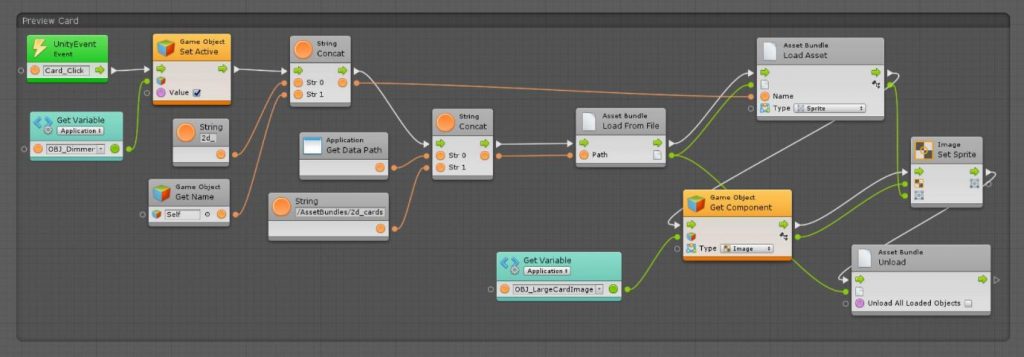
This was the simple asset loading and unloading BOLT graph I put together to help others who were having issues using AssetBundles in BOLT.
NOTE: There is a loading and unloading delay if your asset packing script in Unity is set to compress the assets. It’s best to leave the uncompressed for quick loading.
Tagging Assets to be Bundled
You must tag all of the assets you want in the AssetBundle using “AssetBundle Tag” located in the Preview window of Unity. You can split the assets into multiple bundles to make them load faster.
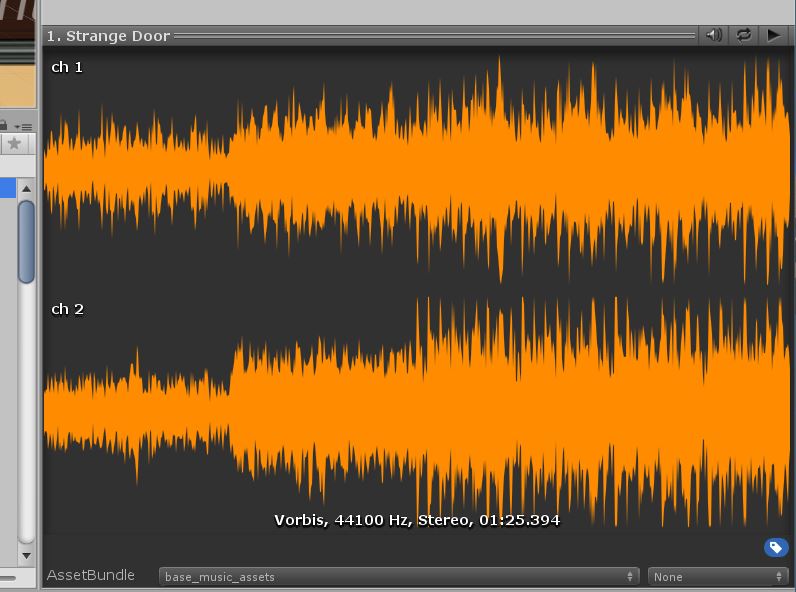
Asset Bundle Building Script
Here is the script needed to build uncompressed AssetBundles in Unity. Create this C# script and put it in the “Editor” directory under “Assets”.
using UnityEditor;
using System.IO;
public class CreateAssetBundles
{
[MenuItem("Assets/Build AssetBundles")]
static void BuildAllAssetBundles()
{
string assetBundleDirectory = "Assets/AssetBundles";
if (!Directory.Exists(assetBundleDirectory))
{
Directory.CreateDirectory(assetBundleDirectory);
}
BuildPipeline.BuildAssetBundles(assetBundleDirectory, BuildAssetBundleOptions.UncompressedAssetBundle, BuildTarget.StandaloneWindows);
}
}
Loading from Multiple Bundles
This is an advanced example of loading from multiple AssetBundles. In this example when a “Play” button is pushed it sets the Playing variable to TRUE and loads the sound file based on the sound name that was stored in a UI Text component. It also gets which AssetBundle the asset should be loaded from using another variable that was set earlier that contains the name of the asset bundle (It matches the AssetBundle Tag) It then takes the location of the App on the computer and STRING Concats everything together to load the assetbundle and then the file needed from inside. Sets the Volume and plays the audio clip that was loaded. It ends by unloading the asset. So this Graph loads the asset from the assetbundle and plays it and then unloads the assetbundle.
